Callbacks!
Goals:
- Use functions as objects, passing them to other functions as callbacks
Coding Snippets:
- The
spiro
function creates a spirograph usingn
repetitions of any shape. It expects the directions for that shape to be passed to it as an argument, which it internally referes to asdrawShape
. - The function containing the instructions expected by
spiro
(in this case,drawSquare
) is known as a callback.
Math and Computer Concepts:
- Functions are a special type of object representing computations to be performed. Because they are objects, we can work with functions much like we would any other data type: they can be assigned to variables, stored in collections such as arrays, and, the focus of this lesson, passed to other functions as arguments.
- A
callback
is a function passed to another for the purpose of being called back, by that other function, at some later time. - Callbacks are an extremely valuable programming tool. In this lesson, we will explore relatively basic examples. But in the next lesson, we will see how they are essential for making our programs interactive, i.e., input data from another source, accept user input, and more.
Activities:
Save each of the following in a folder called Callbacks.
Copy this
starburst
function to a program called Starbursts. Then draw several varieties of starbursts, by defining callbacks that create the pattern to be drawn in each burst, and passing them tostarburst
as theshape
argument.- ArraySort: Every array has a built-in
sort
function. However,sort
is set up to organize the elements of an array lexicographically, which can yield surprising results.For example, the call to
sort
shown here rearranges the original (already sorted!) array of values as follows:Thankfully, users can use callbacks to specify a rule for sorting. This callback should take two arguments, representing potential elements in the array, return a positive number if the first element should come after (i.e., "is bigger than") the second, and a negative number if it should come before the second one. Define two callbacks,
sortRuleAscend
andsortRuleDescend
, to sort an array of numerical values in increasing and decreasing order. MathGraphs: Move one step closer to making your own, personal "Desmos"! Begin by defining a function to draw a set of x and y axes. Then define another function,
plotGraph
, which draws a curve based on a function that is passed to it as a callback. Define several such callbacks, and then pass each one toplotGraph
to add them to your graph.
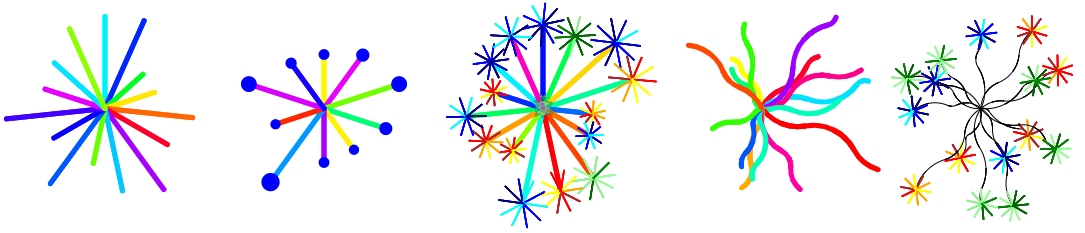