Variables!
Goals:
- Use variables to store values, make code more readable, and to simplify code maintenance.
Coding Snippets:
- The statement
x=0
creates a variable called x that is assigned the value 0. If x already existed, this statement would update x with the value 0. - The statement
x=x+1
would be invalid in math class, but makes perfect sense here. It means: add 1 to the current value of x, then save that result as x. pause
makes the turtle wait the given number of seconds before executing subsequent instructions.- The
cs
function clears all graphics except the turtle.
Math and Computer Concepts:
- Variables are holding tanks for information. These holding tanks are called variables because their content can vary, or change.
- In Coffeescript, as in most coding languages, the equal sign does not test for equality. Rather, it assigns the value of the expression on the right to the variable on the left.
- The examples below illustrate how variables can be used to simplify code and facilitate code maintenance. Both snippets trace out a five-pointed star. However, the code on the right more clearly shows the logic of our work. Additionally, to draw a star with more points, all you need to do is change one number!
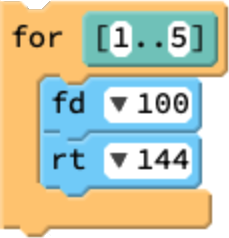
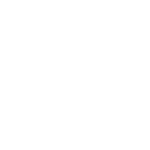
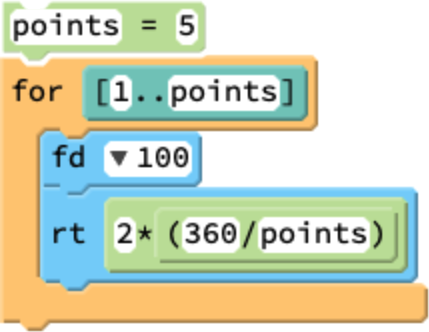
Activities:
Save all programs for today's class in a folder called Variables. Use variables and loops in each activity.
- Code a Polygon program that draws a regular polygon with a random number of sides each time it is run. Use a variable,
sides
, to save this random value (which must be greater than two!) in memory. The number of times you iterate and calculations for turn angles should be based onsides
and not a specific number. - Create a Starburst with random-length spikes, without calling
home
. In each iteration, save the random value using a variable, so you can use it twice—once to draw the spike, and again to return to the center. - Draw a DonutWithSprinkles. Start by using dots as sprinkles, placing them randomly. For a tougher challenge, make "jimmies", i.e., sprinkles in the shape of little lines that point in all directions.
- Draw a HandSpiral that uses
random
to help determine how far the sprite moves and/or turns. But use a variable in the loop so that the turn radius gets bigger with each iteration. - Create a GrassyField, where the blades can vary a bit, randomly. For an added challenge, draw the blades at slight random angles.