Teacher's Guide: Dot Art!
Overview
This first lesson introduces students to the Pencil Code programming environment, addressing drawing basics and file creation and management. Additionally, the lesson introduces some fundamental concepts and terminology, including functions and issues related to syntax and style.
The six basic functions introduced in this section are intuitive. The exercises are intentionally straightforward and designed to give students a chance to be creative and explore. The simplicity of the lesson also frees up the teacher to address other pedagogical concepts, discussed below.
More about the lesson
Setting up
To set up an account, simply navigate to pencilcode.net and select New Account
(in the top right corner). Students' account names should not include any personally identifiable information, such as their real names. This restriction owes to the Children's Online Privacy Protection Rule (COPPA). In short, Pencil Code sidesteps legal issues related to information collection by simply not collecting any personally identifiable information.
In my classes, I record all student usernames and passwords in a spreadsheet. This facilitates viewing their work and also being able to edit it, which sometimes is an expedient way to share feedback rather than communicating it all through email or some other means. Additionally, having a record of passwords is useful for the inevitable forgotten password: because Pencil Code doesn't collect email addresses, there is no automated system to regain a lost password, so having this backup can save the day.
Function arguments
Each of the functions introduced in this lesson takes one or more arguments. There are several aspects of these arguments that should be explored and discussed, though the term argument will not be formally introduced until the next lesson.
For rt
and lt
, students will either guess or quickly discover through experimentation that the argument is measured in degrees. The argument to fd
and bk
, however, is a relative measure, which we might call "units." The blocks in the background grid measure 25 units on a side. Lead students to deduce this feature by instructing them to enter the statement fd 100
, which moves the turtle forward four grid lines.
In terms of terminology, there is little harm in calling the graphical units pixels. Formally, a pixel is a "picture element", which is a physical point in a raster image, and the smallest controllable element of a picture represented on the physical screen. The actual size of a single graphical unit in Pencil Code, however, depends on the browser's zoom setting, but match to pixels when the browser's zoom setting is at 100%.
fd
, bk
, rt
, and lt
can all take negative values as arguments, with predictable results.
speed
Students will quickly express a "need for speed" as their scripts become longer. However, discourage use of speeds greater than 5 until after students have completed debugging, as higher speeds make it difficult to identify mistakes.
When the argument to the speed function is a positive number, it determines the number of turtle moves per second. However, as will be discussed further in the Technicalities section below, because of the way that Pencil Code animation works, increasing the argument only shows a noticeable effect up to about speed 100
. Hence, while students enjoy entering statements such as speed 999999999
, they don't get much extra oomph for all the extra digits.
The speed
function operates on a different basis when passed the argument Infinity
(or, equivalently, 0
or negative values). Superficially, speed Infinity
makes the program process as fast as the computer can compute. However, a greater impact of speed Infinity
is to change the way Pencil Code renders animation, switching from the default queue-based approach to a frame-based approach. The full explanation of this topic will be provided in later lessons, beginning with Add More Turtles. An introduction is also provided in the Pencil Code Reference for Animation.
Block versus text mode
Pencil Code defaults to block mode, but students quickly realize that they can toggle to text mode. As students get to more advanced topics, they will need to use text mode more frequently, as there aren't blocks for all topics. However, even for students who transition to text quickly, blocks remain useful for looking up syntax as new functions and structures are introduced.
Comments
Comments (a.k.a., remarks) are created with a single hash tag, #
. They allow the coder to include material in their code that they can see but that Pencil Code will not process. In block mode, comments are created by pressing Enter
to make a free line, then entering #
.
The lesson describes comments as "notes that we include in our programs", though it stops short of explaining what for. Use comments to add explanations for how your code works, or as section headings to help make the organization of your code more obvious and thereby improve the readability of the code. Additionally, comments can be used for "commenting out" code—i.e., temporarily hiding statements from the computer, without having to delete it. This can be useful for testing out different code designs, for debugging, and more.
Not explicitly shared with students is that pairs of triple hash tags, ###
, can be used to comment out multiple lines of text at once.
In addition to comments, a judicious use of blank lines can also do much to improve the readability of a script.
Thumbnails
"Take pride in your work!" Insist that students save their programs with a thumbnail image. This can be done by saving the file after a program runs and the graphic appears on the screen. If nothing else, a thumbnail can help the teacher find what he or she is looking for when looking through a student's "creatively-named" activities.
Colors
Many students enjoy experimenting with different colors. A list of predefined colors can be found in the Pencil Code colors reference. (This page is easy to find using a web search on "Pencil Code colors".)
In addition to 149 predefined HTML colors, Pencil Code includes a special color-related variable called erase
. This value can be passed in the place of a color in each of the functions leart thus far. For example, dot erase, 50
can be used to cut a hole in the center of a donut, rather than using a dot with a color matching the background.
The background color cannot easily be changed directly using any of the standard Pencil Code functions. A means to control it by using the jQuery that underlies Pencil Code is described in the teachers notes to the Label Recycling! lesson. In the meantime, a simple and intuitive workaround is to use a sufficiently-sized dot to make a solid color background:
dot black, 5000
Notes to activities
An optional challenge for Snowman is to make the dots tangent to each other. Doing so not only highlights the underlying geometry concept, but also promotes thinking algorithmically. The key is to have the turtle move half the diameter of the first circle and half the diameter of the second—but let students figure that out for themselves. Aside from serving as a good problem-solving activity, this challenge can be a good reinforcement of (or potentially an authentic opportunity to introduce) math concepts of diameter, radius, and, of course, the property of tangency.
Moreover, in this and in subsequent activities, discourage "guess and check" approaches, in favor of thinking through the underlying math. Using math to inform our coding will come in handy in many of our subsequent lessons, especially as students progress to the use of variables, such as in iterative situations, or, later on, when defining functions of their own.
Additional activities
- DotIllusion: Use dots to create this optical illusion. (The center dot in each cluster of dots is the same size.)
- DotMessage: Using only dots, write out a simple message.
- DotSteps: Create the illusion of footsteps by varying the turtle speed. Using only the functions introduced in this lesson, make the turtle appear to jump instantaneously from point to point (i.e., with speed set to
Infinity
), but carry out each call todot
atspeed 1
.
Beyond the lesson
Source code
To see the source code for any of the examples provided in the lesson, change the word home
to edit
in the URL after clicking on the link. For example, clicking on the Cookie image in the lesson leads to
https://hacker.pencilcode.net/home/01-DotArt/Cookie
Whereas the following shows the code as well:
https://hacker.pencilcode.net/edit/01-DotArt/Cookie
Naming Conventions: CamelCase
Students benefit from guidance on approaches to naming and organizing their work, and there is no better place to start than with the first lesson. An important aspect of organization is choosing good names for folders and files (and also variables, which will be introduced in a later lesson).
This lesson does not explicitly address how to create a Pencil Code file, as it is obvious. However, the first activity implicitly instructs students to use CamelCase: "You know UPPER CASE and you know lower case. CamelCase is in between, with humps".
In addition to CamelCase, two other styles for combine multiple words in a single name are kebab-case and snake_case. The former style appears frequently in CSS (it is not used in JavaScript because the dash is parsed as a minus sign); the latter style is typically used in JavaScript for indicating constants, which by convention are written in all-caps, e.g., EXAMPLE_CONSTANT_NAME.
Keyboard shortcuts
Students may notice from a mouse-over of the play icon that CTRL+enter
(PC) or Command+enter
(Mac) will run the program. Though probably premature for students at this point, another useful Pencil Code keyboard shortcut is CTRL+/
(PC) or Command+/
(Mac) to comment out all currently-selected lines of text. Pencil Code provides several other keyboard shortcuts, which are listed in the Pencil Code Reference on Shortcuts.
Test panel
In the bottom right-hand corner of the coding environment is a Test Panel comprised of a command line and console. Output from the see
function (a.k.a., debug
or console.log
) appears in the console. Single-line entries can be entered in the command line. Statements entered here affect the current graphical window, but are not saved. Enter the name of a Pencil Code function at the command line, without an argument or parentheses, to obtain a brief explanation of usage. Entering help
provides a list of functions for which such documentation is available.
What can go wrong
Logging in
Students sometimes report log-in difficulties when they attempt to sign in to their accounts from the main Pencil Code screen. If this happens, instruct them to navigate to their own page (e.g., StudentAccount.pencilcode.net) and log in from there.
Naming/renaming files
Recall that folders are created by naming a file and a folder at the same time. For the Dot Art! lesson, a common early mistake is to save a program asDotArt
, rather than saving that program in a folder named DotArt
. Renaming in this case becomes a stumbling block, as it involves a two-step process.
For example, suppose one creates a program named Folder
:
The user cannot subsequently change the name of the file called Folder
to Folder/Filename
in one step. First, one must rename Folder
as something else (perhaps Filename
). Doing so frees up the name Folder
to be used as a folder name, allowing the user to subsequently rename Filename
as desired, i.e., Folder/Filename
.
Syntax errors
In the early lessons, and especially when using only blocks, the topic of syntax may seem a long way off. However, many students will soon navigate to the text-based version of the editor. While CoffeeScript syntax is more forgiving than most, it must nonetheless be adhered to correctly.For students who have adopted text-based programming, a common mistake in this lesson is omitting a comma between two arguments in a function call, such as
dot blue 100
Thankfully, Pencil Code provides an effective error prompt in this case:
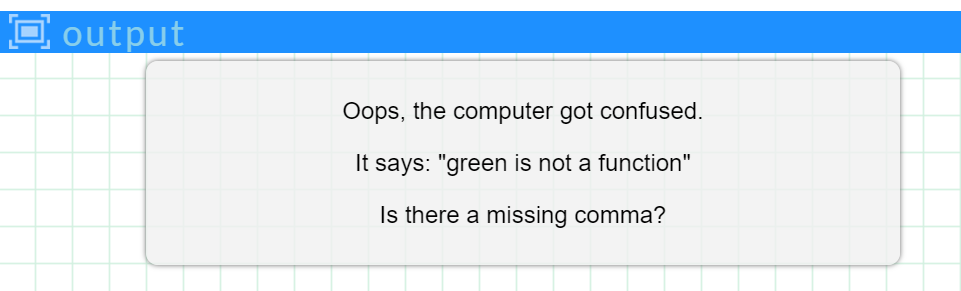
Pedagogy
The activities in this lesson may strike some students as trivial, especially for students with some past programming experience. Share with them that a primary objective of this lesson is to get familiar with the Pencil Code environment and file management, and emphasize that more generally they need to get requisite practice with new material before moving on. Coding, like mathematics, is a cumulative subject, and it will get sufficiently difficult soon enough! Learning to code has less to do with learning about new functions and structures than it does with developing computational thinking skills, i.e., breaking down problems and solving them with algorithms. Creativity also plays a big role in designing and implementing meaningful programs. Finally, good coding style and organizational skills are emphasized too, which become all the more important as students embark on more complicated and longer programs. For example, comments are introduced in the first lesson, as are naming conventions. Students need to spend adequate time applying and reinforcing these concepts before moving on. Headaches with organization are good to have earlier than later, because it's easier to fix problems before students have lots of files.
To support the development of computational thinking skills and to ensure sufficient practice with new concepts, I insist that students only use the Pencil Code features which have been formally introduced in the current or previous lessons. Challenge your students: Sure, they can make a simple snowman using dots. But can they add a convincing carrot nose, arms, a stove-pipe hat, or other features using just dots? And, what other type of art can they come up with, using only dots? Being restricted to a limited palette of tools presents a special challenge. The panda, horse, and cookie shown on the sheet were my creations; what can your students come up with?
While I prohibit the use of "coding snippets" not yet introduced, to ensure that students truly explore and master the current tools, students are welcome to explore on their own outside of class (not just because I can't stop them, but because exploration is a good thing). However, I make clear that I don't provide assistance on those concepts until we reach them in the syllabus. I encourage students to make a MyStuff folder for work their extracurricular scripts, and they are free to explore other concepts to their hearts content there. I can't keep them from working ahead in this way. I love when they put in extra time outside of class, but I want to channel that energy as much as possible into continuing to develop master of the current set of concepts.
Technicalities
CoffeeScript
CoffeeScript has a one-to-one mapping to JavaScript. In fact, before a CoffeeScript program is run, it must be compiled into JavaScript, which is then interpreted by the browser. The Pencil Code environment does this all behind the scenes when a coder presses the play button.
Coding terminology
A strong effort has been made to be consistent, as well as accurate, in use of terminology. For example, the term coding snippets is used because the code introduced under that lesson heading can be a mix of functions, expressions, operators, and so on. No other catch-all phrase really seems to fit.
While one goal is to be consistent and accurate, another is to avoid being pedantic. In this unit, the more experienced coder may have caught the abuse of the term function to describe rt
, dot
, and so on. These are, in fact, methods of the Turtle
object, which are operating on the default instance of Turtle
, pointed to by the variable turtle
.
The reason for choosing the term function is that the distinction has no value to students at this point, as they are not yet familiar with object-oriented programming concepts at this point. Moreover, for the younger audience, the mathematical concept of a function is likely still relatively new to them, if they have learned it at all. Thus, using the term can be a good reinforcement as well as a concept to build on.