Teacher's Guide: Custom-Sized Sprites!
Overview
The focus of this lesson is how to set the size of a custom sprite using a string argument. Additionally, this lesson provides continued opportunity to reinforce skills with previously-learned functions and concepts and further contributes to the development of computational thinking skills.
More about the lesson
Sprite
constructor syntax
Creating sprites with sizes larger or smaller than the default is straightforward. The key is simply to follow the Sprite
constructor syntax correctly. This entails (1) specifying the color first and then the dimensions; (2) using a lower-case x
and not an *
or X
between the desired width and height; and (3) not putting any spaces between the dimensions and the x
.
Strings
The string data type is explicitly discussed because of its use as an argument to the Sprite
constructor. For novice coders, the term "string" may not be not intuitive. Take a moment to reflect on the (apparent) etymology of the term: a string is "(zero or more) characters strung together (in order) as a single unit of data".
Students have been making use of strings from day one in this course. The predefined Pencil Code color variables all point to strings, as do "special colors" such as path
, erase
, and transparent
. One can demonstrate this using the see
function in the command line of the test panel, but arguably more straightforward and convincing is a demonstration that pen
"red"
works the same as pen
red
. Strings are ubiquitous and play a hugely important role in programming. They will be revisited in Add Text! and discussed in greater depth in the associated teachers notes to that lesson.
Notes to activities
The OddBlocks allows for a lot of creativity and variation. Also, though sprites are all fundamentally rectangular, we can use the tools from the previous lesson to create "blocks" in other shapes that fit those together, including triangles and even circle-based shapes. Popular program variants focus on different designs, such as Flags and Minecraft characters, or on enhanced animations.
For many students, the logic behind RingsInMotion takes some work. Arguably the simplest approach is to draw off-centered circles on the sprites, i.e., with one edge of the circle on the center of the sprite, and then rotate them with simple calls to rt
or lt
. Alternatively, students could create sprites with rings centered on them, and then use the turn functions with two arguments to make them follow a circular path (but giving the illustion of rotating).
Additional activities
- Create a custom sprite in the shape of a Rocket, positioning the "tailpipe" at the center of the sprite's canvas, so that using
pen
you can easily draw smoke coming out of the rocket in the right position. As an extra challenge, encourage students to explore use of variables to make the rocket appear to accelerate, rather than move at constant speed. - Simulate a SolarEclipse, using sprites for the sun and the moon. Move the moon across the screen; as it passes over the sun, the background should get gradually darker. For an extra challenge, make stars appear as the sky gets dark enough.
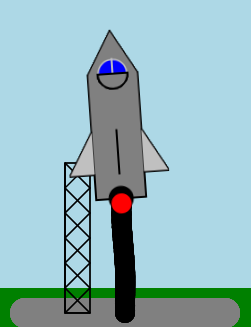
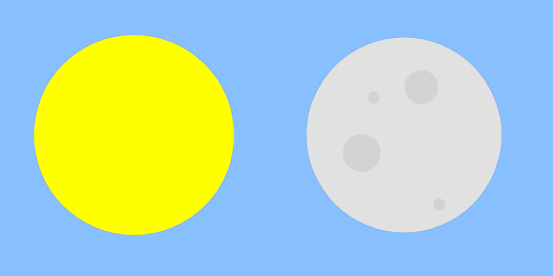
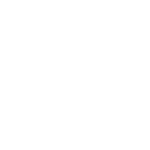
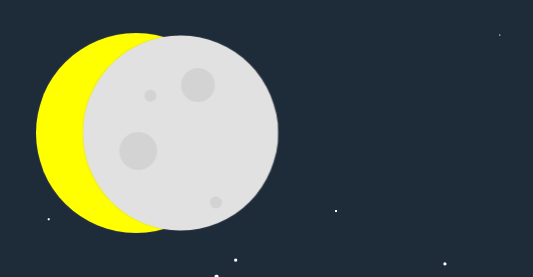
Beyond the lesson
Passing objects to the Sprite
constructor
The Sprite constructor accepts a single argument. In this lesson, students are shown the explicit use of a string as an argument, e.g., "red 500x500"
. Students likely will not have realized previously that when they specified a color they were in fact using a variable that referenced a string (i.e., the variable red points to the string "red"). So, the difference here is that the string passed as the argument to the Sprite
constructor is simply a more information-rich variant of what was used before.
In addition to strings, the Sprite
constructor also accepts objects as an argument. Objects are one of the seven underlying data types in JavaScript, and will be addressed in greater detail beginning in the Date Objects! lesson. However, students may stumble across the object notation prior to that lesson, such as from examples they find elsewhere on the Pencil Code website.
The following provides a simple example of using an object to create a sprite in the shape of a green rectangle, equivalent to the statement g = new Sprite("green 100x200")
:
The object syntax illustrated here is JavaScript. CoffeeScript allows us to simplify it, and as a result the same code may appear in a variety of guises. Some of the possibilities are illustrated here. Objects, including syntax variants, will be explored in depth in Custom(ized) Objects!.
One benefit of object notation is that it permits the coder to specify a wider array of sprite properties. For example, students may notice that the order in which sprites are created by default determines which is on top. The notes to the Images! lesson describe how to use the CSS zImage
property to override the default ordering.
Sprite re-sizing
The grow
and scale
functions permit sprite resizing. grow
simply changes the size of the sprite. scale
also changes the size of the sprite, but also changes the effect of all functions that depend on a measure of scale as well, such as movements and pen width. An illustration of these effects is provided in the following script:
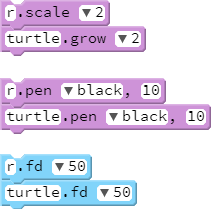
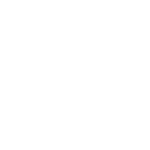
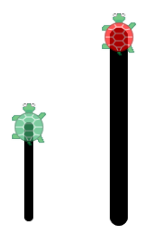
Calls to grow
and scale
are cumulative. Thus, two calls to grow 2
have the same effect as grow 4
, and grow 0.5
offsets a prior call to grow 2
.
Note that calls to home
also reset many turtle properties to their original settings. This includes undoing the effects of grow
and scale
.
Additionally, sprites have methods width
and height
which can be used to access current dimensions (when called without an argument) or to modify one of the two dimensions separately (when passed a whole number representing number of pixels). The following code provides an alternative means to double the size of a sprite s
:
s.width(2*s.width()) s.height(2*s.height())
Resizing a sprite in only one dimension is an effective way to create an oval, as this script illustrates.
What can go wrong
Invalid syntax
The main pitfalls to watch out for in this lesson involve syntax. Students will likely miss one of the details in the string argument to the Sprite
constructor, such as omitting one or both quotes, or using something other than a lower-case x
for the times sign.
One of the challenges to this lesson is that mistakes to the string argument will lead to unexpected results, though not necessarily cause the program to crash or otherwise yield an error message. For example, the statement t = new Sprite("red 100x100")
yields the following image:
This is not a bug, but rather an unintended result from a built-in serach feature of the Sprite
constructor. As described in the Images! lesson, when Pencil Code cannot parse the string argument in terms of color and/or dimension, it will search a catalog of free images for an image that matches that string.
Invalid dimensions
Sprite dimensions must be specified as counting numbers, because they specify the number of pixels used for the underlying image on the HTML <canvas>
element. The activities in this lesson are unlikely to give rise to this error. It is more likely to arise if a sprite were created based on numerical calculations, such as based on the size of the visible screen (as described in Array Destructuring!). In such cases, the JavaScript round
, ceil
, or floor
functions would likely prove useful.